Voice Assistant using Python
- I am Saheer Ahmed from Web development team, OWASP Student Chapter.
- I have written the program inspired by my favourite character Iron man's Artificial Intelligence "Jarvis".
- I first started writing code for the information of colleges and their NIRF Rankings for students.
- Later on I also started making it for the information of devices which are helpful for students and information on special student discounts like Apple Student Program, Samsung Student Program.
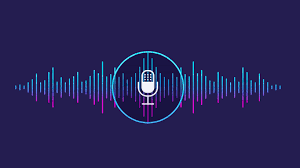
Let's first start with the installation of modules for the required program: (In terminal type the following things)
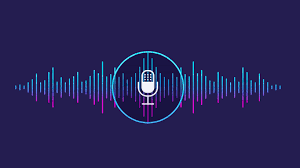
- pip install pyttsx3
In case you receive such errors:
- No module named win32com.client
- No module named win32
- No module named win32api
- pip install pypiwin32
- pip install speechRecognition
- import speechRecognition as sr
Defining Task 1: To search something on Wikipedia
To do Wikipedia searches, we need to install and import the Wikipedia module into our program. Type the below command to install the Wikipedia module :
- pip install wikipedia
- import datetime
What is sapi5?
- Microsoft developed speech API.
- Helps in synthesis and recognition of voice.
What Is VoiceId?
- Voice id helps us to select different voices.
- voice[0].id = Male voice
- voice[1].id = Female voice
Writing Our speak() Function :
We made a function called speak() at the starting of this tutorial. Now, we will write our speak() function to convert our text to speech.
def speak(audio):
engine.say(audio)
engine.runAndWait() #Without this command, speech will not be audible to us.
Let's now jump to the code:
import pyttsx3
import speech_recognition as sr
import datetime
import wikipedia
import webbrowser
import os
engine = pyttsx3.init('sapi5')
voices = engine.getProperty('voices')
engine.setProperty('voice', voices[0].id)
def speak(audio):
engine.say(audio)
engine.runAndWait()
def wishMe():
hour = int(datetime.datetime.now().hour)
if hour>=0 and hour<12:
speak("Good Morning!")
elif hour>=12 and hour<18:
speak("Good Afternoon!")
else:
speak("Good Evening!")
speak("Jarvis for your help Sir")
def takeCommand():
#It takes microphone input from the user and returns string output
r = sr.Recognizer()
with sr.Microphone() as source:
print("Listening...")
r.pause_threshold = 1
audio = r.listen(source)
try:
print("Recognising...")
query = r.recognize_google(audio, language='en-in')
print(f"User said: {query}\n")
except Exception as E:
# print(E)
speak("I can't hear it sir")
print("Say that again please...")
return "None"
return query
if __name__ == "__main__":
wishMe()
# while True:
if 1:
query = takeCommand().lower()
# Logic for executing tasks based on query
if 'wikipedia' in query:
speak('Searching Wikipedia...')
query = query.replace("wikipedia", "")
results = wikipedia.summary(query, sentences=2)
speak("According to Wikipedia")
print(results)
speak(results)
elif 'open youtube' in query:
webbrowser.open("youtube.com")
elif 'open google' in query:
webbrowser.open("google.com")
elif 'open stackoverflow' in query:
webbrowser.open("stackoverflow.com")
elif 'play music' in query:
music_dir = 'D:\\Non Critical\\songs\\My playlist'
songs = os.listdir(music_dir)
print(songs)
os.startfile(os.path.join(music_dir, songs[0]))
elif 'the time' in query:
strTime = datetime.datetime.now().strftime("%H:%M:%S")
speak(f"Sir, the time is {strTime}")
elif 'open code' in query:
codePath = "C:\\Users\\SHAIK SAHEER AHMED\\AppData\\Local\\Programs\\Microsoft VS Code\\Code.exe"
os.startfile(codePath)
elif 'open facebook' in query:
webbrowser.open("facebook.com")
elif 'open twitter' in query:
webbrowser.open("twitter.com")
elif 'open whatsapp' in query:
webbrowser.open("whatsapp.com")
elif 'open instagram' in query:
webbrowser.open("instagram.com")
elif 'open wikipedia' in query:
webbrowser.open("wikipedia.com")
elif 'open google map' in query:
webbrowser.open("google.com/maps")
elif 'open car dekho' in query:
webbrowser.open("www.cardekho.com")
elif 'open cars 24' in query:
webbrowser.open("www.cars24.com")
elif 'open dude perfect' in query:
webbrowser.open("dudeperfect.com")
elif 'open etv' in query:
webbrowser.open("www.etv.co.in")
elif 'open amazon' in query:
webbrowser.open("www.amazon.in")
elif 'open zoom' in query:
webbrowser.open("zoom.us")
elif 'open flipkart' in query:
webbrowser.open("flipkart.com")
- This is the code and path is added on my device for the command "open code".
- You can add you own path by copying and pasting.
- To open links you can run the program and can search just by speaking.
- I have added English(Indian) for easy recognition and used google as browser to search.
- You can add much more to this like I have added the things as mentioned in the introduction.
0 Comments